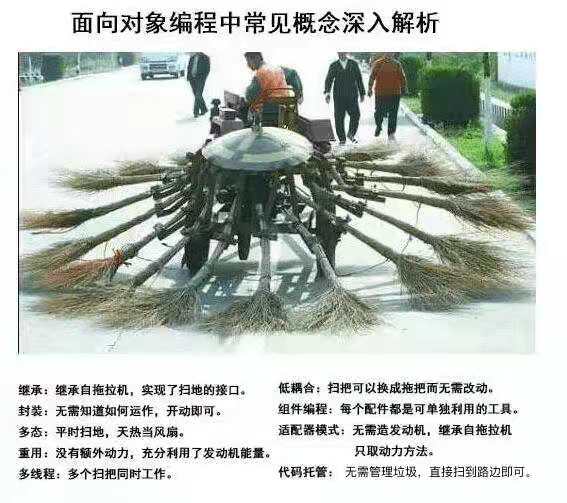
import sun.misc.BASE64Decoder;
import sun.misc.BASE64Encoder;
import java.io.UnsupportedEncodingException;
import java.util.Base64;
public class Base64Util {
/**
* String转换为Bse64
* @param str
* @return
*/
public static String strConvertBase(String str) {
if(null != str){
Base64.Encoder encoder = Base64.getEncoder();
return encoder.encodeToString(str.getBytes());
}
return null;
}
/**
* Base64转换成String
* @param str
* @return
*/
public static String baseConvertStr(String str) {
if(null != str){
Base64.Decoder decoder = Base64.getDecoder();
try {
return new String(decoder.decode(str.getBytes()), "UTF-8");
} catch (UnsupportedEncodingException e) {
return null;
}
}
return null;
}
/**
* base 64 encode
*
* @param bytes
* 待编码的byte[]
* @return 编码后的base 64 code
*/
public static String base64Encode(byte[] bytes) {
return new BASE64Encoder().encode(bytes);
}
/**
* base 64 decode
*
* @param base64Code
* 待解码的base 64 code
* @return 解码后的byte[]
* @throws Exception
*/
public static byte[] base64Decode(String base64Code) throws Exception {
return new BASE64Decoder().decodeBuffer(base64Code);
}
}